How To Use Pyqt Signals And Slots
- Table of Contents
- The concept of signals and slots
- Connecting with signals and slots
- Disconnecting
- A parser-formatter using signals and slots
- Conclusion
Both the rate and the setRate methods can be connected to, since any Python callable can be used as a slot. If the rate is changed, we update the private rate value and emit a custom rateChanged signal, giving the new rate as a parameter. We have also used the faster short-circuit syntax. Events and signals, on the other hand, are central to PyQt. Signals and slots are used to connect one object to another. An example is the perennial pushbutton, whose clicked signal gets connected to the accept slot function of a dialog box. Signals are used to connect entities internal to the application.
The concept of signals and slots is possibly the most interesting innovation in the Qt library. Good widgets and a clean API are rare, but not unique. But until Qt appeared on the horizon, connecting your widgets with your application and your data was a nasty and error-prone endeavor — even in Python. I will first discuss the problem that is solved by signals and slots in some detail; then I will introduce the actual mechanics of the signal/slot mechanism, and finish with an application of the technique outside the GUI domain.
The problem in a nutshell: imagine you have an application, and the application shows a button on screen. Whenever the button is pressed, you want a function in your application to execute. Of course, you'd prefer that the button doesn't know much about the application, or you would have to write a different button for each application. In the next example, the button has been coded to work only with an application that has the
Example 7-1. A stupid button which is not reusable
CallbacksThis is no solution— the button code isn't reusable at all. A better solution would be to pass the
Example 7-2. A simple callback system
How To Use Pyqt Signals And Slots Games
Using
This is useful because
This is far less flexible. In the previous example, we created an argument tuple to be passed to the
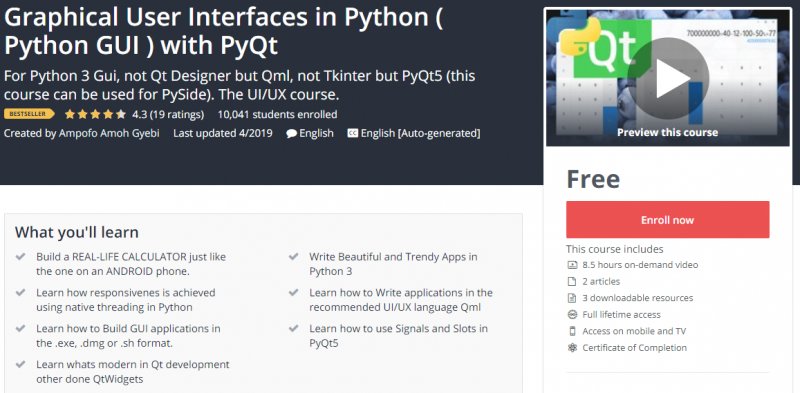
In more recent versions of Python, you don't need to use
That is, when calling the constructor of the superclass, you can pass

Unfortunately, this callback system is not quite generic enough. For example, what if you wanted to activate two functions when the button is pressed? While this is not likely in the simple example, under more complex situations it often occurs. Think of a text editor where editing the text should change the internal representation of the document, the word count in the statusbar, and the edited-indicator in the titlebar. You wouldn't want to put all this functionality in one function, but it is a natural fit for signals and slots. You could have one signal,
Wouldn't it be extremely comfortable to simply have a central registry where interested parties could come together? Something like:
Example 7-3. A central registry of connected widgets
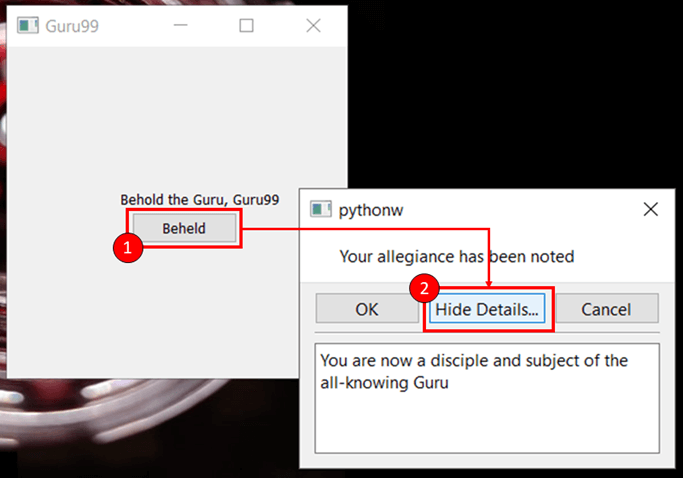
This is one step up from the previous example, which was an extremely crude implementation of the well known Observer design pattern, in that there is now a ‘neutral' object that mediates between the button and the application. However, it is still not particularly sophisticated. It certainly wouldn't do for a real application — where there might be many objects with the same ‘occasion'.
Pyqt Signals And Slots
It is quite possible to implement a solution like this in pure Python, especially with the weak references module that debuted in Python 2.1. Bernhard Herzog has done so in his fine Python application
We've just outlined the problem which the developers of Qt at Trolltech have solved in a unique and flexible manner. They created the concept of signals and slots.
On the whole the concept is really neat and clean and the implementation well-executed. What's more, the concept is even better suited to Python than it is to C++. If you want to use signals and slots in C++, you have to work with a preprocessor, called
Signals and slots are not magic, of course. As with our simple Python registry, there has to be a registry of objects that are interested in signals. This registry is updated by the
In a nutshell, signals and slots are the solution Qt provides for situations in which you want two objects to interact, while keeping that fact hidden from them.
Signals, messages, events: This is one area where there is a perfect Babel of tongues. Even really knowledgeable people like Dr Dobbs' Al Stevens get confused when confronted with terms like ‘message', ‘event' or ‘signal'.
In PyQt programming, the term '‘message' is quite irrelevant — it is used in Windows programming to indicate function calls made from your application to the Windows GUI libraries.

Events and signals, on the other hand, are central to PyQt. Signals and slots are used to connect one object to another. An example is the perennial pushbutton, whose
How To Use Pyqt Signals And Slots Using
Events are more often generated directly by user input, such as moving or clicking with the mouse, or typing at the keyboard. As such, they don't connect two class instances, but rather a physical object, such as a keyboard, with an application. Events are encapsulated by the